JavaScript & TypeScript classes
An high-level introduction to classes in JS and TS
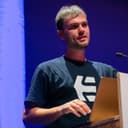
Fabio Biondi
Google Expert | Angular
# Introduction
This article briefly introduces classes in both JavaScript and TypeScript, providing a foundational understanding for future lessons, in which we will explore classes in greater detail.
While we won't cover advanced or nuanced features of classes at this time, this overview is designed to help you understand their basic structure and purpose.
Classes in Modern JS ecosystem
It's also worth noting that while classes offer a familiar object-oriented approach, they aren't as commonly used in the JavaScript ecosystem today.
Many developers opt for functional programming patterns and hooks, especially with modern frameworks like React.
Angular still use classes to defined its Components, Directives and several other features of the framework are managed by classes but in the latest versions many of these features can now be written as functions instead.
However, knowing how classes work will still be valuable for certain use cases and when reading or maintaining legacy code.
# Classes in JavaScript
JavaScript introduced classes in ECMAScript 2015 (ES6), and they act as syntactic sugar over JavaScript's existing prototype-based inheritance. While they look more like traditional classes found in other languages (like Java or C#), they still follow the same prototypal inheritance under the hood.
Although the examples are very simple, we assume that the reader already has some OOP basics and knows the concept of classes and instances
# Basic Class
Let's start with a Simple Class in JavaScript
-
Constructor Method: The constructor
method is a special method used for initializing objects created with a class. In our Person
class, the constructor accepts two parameters (name
and age
) and assigns them to the instance being created.
-
Methods: The greet()
method is defined within the class and is available to all instances of the Person
class. This method logs a message using the instance's properties (this.name
and this.age
).
-
Instance Creation: We use the new
keyword to create an instance of the class, passing in values for the constructor
. In this case, person1
is an instance of the Person
class.
# Inheritance in JavaScript Classes
Classes in JavaScript can also be extended, allowing us to create new classes that inherit properties and methods from a parent class.
-
extends
Keyword: The Dog
class extends the Animal
class, meaning it inherits its properties and methods. However, we override the speak()
method in Dog
to provide custom behavior (barking).
-
Super Constructor: When we use inheritance, we can call the parent class's constructor using the super()
function. In this example, Dog
implicitly calls Animal
's constructor when we create a new instance of Dog.
# Classes in TypeScript
TypeScript builds on JavaScript's class system by adding static typing, access modifiers, and other features to enhance object-oriented programming.
-
Type Annotations: In TypeScript, we specify the types of properties and method parameters. For example, name
is of type string
, and age
is of type number
. This ensures that when we create instances of Person
, we pass in the correct data types, reducing potential runtime errors.
-
Return Type: The greet()
method specifies a return type of void
, indicating that it doesn't return any value.
# Access Modifiers in TypeScript
TypeScript introduces access modifiers (public
, private
, protected
) to control the visibility of class members.
-
public
Modifier: By default, class members are public, meaning they can be accessed anywhere.
In this example, name is public
and can be accessed outside the class.
-
private
Modifier: The salary
property and getSalary()
method are marked as private
, which means they can only be accessed within the class itself.
Any attempt to access them from outside the class results in a compilation error.
# Static Methods and Properties
Both JavaScript and TypeScript support static methods and properties, which belong to the class itself rather than to any instance of the class.
static
Members: The pi
property and calculateArea()
method are marked with the static
keyword, meaning we can access them directly from the class without creating an instance.
Static members are useful when we want to associate utility functions or constants with the class itself.
# Conclusion
Classes in both JavaScript and TypeScript provide a powerful way to organize and structure our code, making it more modular, reusable, and maintainable.
With TypeScript's type annotations, access modifiers, and additional features, we can write more robust and predictable applications.
Accedi a TUTTI I CORSI PREMIUM ad un unico prezzo!