Destructuring
Understanding Destructuring in TypeScript
A simple and useful way to handle variables
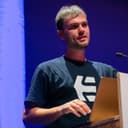
Fabio Biondi
Google Expert | Angular
# Intro
Destructuring in TypeScript is a powerful feature that enables you to extract values from arrays, objects, and other data structures into separate variables.
This approach offers a concise and expressive method for handling complex data types, enhancing readability, and reducing code verbosity.
# Array Destructuring
Array destructuring lets you unpack values from arrays into variables using a syntax that resembles array literals.
In this example, first
and second
variables capture the first two elements of the numbers array, while the rest
variable uses the rest operator (...
) to gather the remaining elements into a new array.
# Object Destructuring
Object destructuring allows you to extract properties from objects and assign them to variables with matching names.
When you initialize a variable with a value, TypeScript infers the type of that variable from the assigned value. This is why we don't need to specify the type for person
explicitly and why type checking still works.
You can also define default values when properties are not defined, avoid if
and manual assignments:
Here, variables firstName
, age
, and city
are assigned the corresponding values from the person object, or the default values.
# Function Parameter Destructuring
Destructuring can also be used in function parameters to directly extract values from objects or arrays passed as arguments.
In the following examples we have a printDetails
function that accept an object of type Person
and then we display its property using the syntax person.propName
:
This feature is widely used in React components
Destructuring inside the function
Here a updated version that uses destructuring, to assign default values too:
Tip: rename properties
You can also rename properties using the following syntax, propName: newName
:
With Function parameters destructuring
The next function generates the same result but it takes an object as a parameter and directly extracts firstName and age properties from it for use within the function body.
TypeScript Tip: inline vs alias type
In the following snippet, the type of the parameter is defined inline as an object with firstName
and age
properties.
For small functions or one-off cases, defining the type inline can make the code more concise and easier to read.
Anyway, defining an interface for the object can make the code more readable and reusable, especially if the type is used in multiple places but this is good alternative for types used once in the application:
# Nested Destructuring
Destructuring can be nested to extract values from nested objects or arrays.
Here, math
and science
properties are extracted from the nested scores
object within the student
object.
Destructuring in TypeScript offers a concise and expressive way to work with data structures, improving code readability and maintainability.
By leveraging array and object destructuring, you can write more elegant and efficient code.
Accedi a TUTTI I CORSI PREMIUM ad un unico prezzo!