Promises
Asynchronous operations with JavaScript Promises
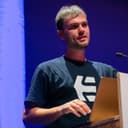
Fabio Biondi
Google Expert | Angular
# Asynchronous Operations
When working with JavaScript, one common challenge is handling asynchronous operations / tasks that don’t complete immediately, like fetching data from a server or performing a time-based operation.
A typical mistake developers make when starting with asynchronous code is trying to return values directly from functions involving asynchronous operations, like setTimeout
or API requests.
Take a look at the following script.
At first glance, you might expect the function getData()
to return 123
after 2 seconds. However, this doesn't work because setTimeout
is asynchronous, meaning the code outside of it (like console.log(getData()))
runs before the timeout callback finishes executing.
The issue here is that the getData
function does not return the expected value 123
because setTimeout
works asynchronously.
The function getData()
itself immediately returns undefined
(since setTimeout
doesn't return anything), while the value 123
is returned inside the callback after 2 seconds, but there's no mechanism to pass it back to the caller.
# Promise
When working with asynchronous operations like setTimeout
or data fetching, we need a "promise" to handle the delayed result.
A promise allows you to return a value at some future point in time after the asynchronous operation completes.
We can wrap setTimeout
in a Promise
so that getData()
can return the result when the timeout
finishes:
- The Promise constructor takes a function with two arguments:
resolve
and reject
.
- In this case, after 2 seconds,
resolve(123)
is called, which returns 123
to the caller.
- The caller can use
.then()
to handle the result when the promise resolves.
This ensures that the function can handle asynchronous operations properly:
If the promise invoke the reject
function, the caller can handle it using catch
:
# Promise in JavaScript Classes
Of course, we can use promises in classes to:
# Conclusion
Using promises with asynchronous operations, for example using fetch
, is extremely useful because fetch is inherently asynchronous and works similarly to how setTimeout
behaves in the previous example.
fetch
is a built-in JavaScript function that makes asynchronous HTTP requests to retrieve or send data to a server, returning a promise that resolves with the response.
Accedi a TUTTI I CORSI PREMIUM ad un unico prezzo!