Work with JavaScript Modules
Import & Export modules, barrel files, dynamic loading & tips
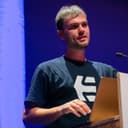
Fabio Biondi
Google Expert | Angular
# Introduction
In modern JavaScript (ES6 and later), modules enable you to break your code into separate files, which enhances maintainability, reusability, and organization.
A module is simply a .js
or .ts
file that exports variables, functions, classes, or objects, allowing you to import and use them in other modules.
There are two core concepts to understand:
-
Exporting: This process makes certain parts of a module—such as variables, functions, and classes—available for use in other files. You can export items using named exports or a default export, as we will explore later in this lesson.
-
Importing: This is how you bring functionality from another module into your current module, allowing you to use its exported values. By using modules, you can decompose complex codebases into smaller, focused components, making it easier to manage and scale your applications.
# Export functions and variables
In this script, utils.ts
uses named exports to export constants and functions, in order to make them to be individually imported into other files.
In index.ts
, the import statement brings in these named exports (multiply
, divide
, and PI
) from utils.ts
, making them available for use.
# Export as default
In JavaScript, a default
export is used to export a single value from a module.
Unlike named exports, you don't need to import it by the same name and it can be imported with any name you choose.
In fact, the following snippet export the multiply
function but it's imported with another name:
# Rename imports
We can also rename named exports using the as
syntax.
In the following snippet you can see how we use different names for both, default and named exports:
# Export arrow function as default
Accedi a TUTTI I CORSI PREMIUM ad un unico prezzo!