Arrow Functions
The differences between regular and arrow functions
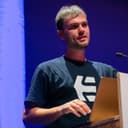
Fabio Biondi
Google Expert | Angular
# Regular Function in TypeScript
Here's a simple TypeScript function called add
that takes two numbers as arguments (a
and b
) and returns their sum.
The type annotations ensure that both inputs and the return value are numbers.
In the example, we call add(3, 4)
, which outputs 7
in the console.
# function declaration vs expression
In the previous example, the following line is a function declaration:
This type of function can be called before it’s defined because it’s hoisted to the top of its scope during the compilation phase.
The following example is a function expression:
The function is assigned to a constant variable.
It’s not hoisted, so it can only be called after the line where it's defined.
# Arrow functions
Next snippet uses an arrow function (=>
), which is a more concise way to write functions in TypeScript (or JavaScript). The key differences from a regular function expression are:
-
Lexical this binding: Arrow functions don't have their own this
, instead, they inherit this
from their surrounding context (you can find more info and examples about this topic at the end of the article, where we use this
inside classes)
-
Syntax: Arrow functions provide a shorter syntax.
# Implicit return
In this version, the arrow function has been simplified using implicit return
, which means that when the function body contains only a single expression, the result of that expression is automatically returned without needing the return
keyword or curly braces.
Functionally, they are identical, but the implicit return
makes the code more concise.
# Implicit type annotation
In the previous version we have explicitly defined the type of return
, which must be number
.
Explicit typing provides more clarity and safety by explicitly defining both parameter and return types.
Next snippet uses implicit type annotation.
This snippet relies on TypeScript's type inference system to deduce the types, which can make the code shorter but might be less clear in more complex scenarios:
If you try the script in the playground and move your mouse over the keyword add
, you will see that the return type will be number
, even if we have not specified it:
# Defaults
In this example, default parameters are used, which allow the function to assign a default value to a parameter if no value is provided when the function is called.
# Return array
Functions can returns any type of data.
Here an example where we return an array:
And it works with inline functions too:
# Return Object
But what if we want to return an object?
The result is not so obvious!
First define a function that returns an object. This works fine, as expected:
Accedi a TUTTI I CORSI PREMIUM ad un unico prezzo!