React Components & TypeScript
A quick introduction to React Components in TypeScript
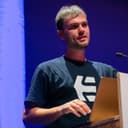
Fabio Biondi
Google Expert | Angular
# Import Components
This example demonstrates basic component creation, importing, and composition in a React application using TypeScript.
We define two React components using TypeScript, Panel
and App
, and demonstrate how these components are used together in a React application.
The use of reusable components is a core aspect of building modular and maintainable React applications.
-
App
component imports the Panel
component using the named import syntax (import { Panel } from './Panel';
).
This allows the App
component to use the Panel
component defined in the Panel.tsx
file.
-
App
Component uses the Panel
component three times, resulting in three components being rendered.
# Export as default
& renaming
In the next example you can see how we use imports and exports in a React application to import and rename two components, Panel
and Card
(which is exported as default
):
When the component is exported as default
we don't need curly braces and component can be easily renamed.
# Pass data to components (properties)
The previous Panel
component was static, displaying the same text for each instance.
Now we update the Panel
component to become dynamic, relying on the title
prop passed to it, demonstrating how to use props to change component behavior and rendering.
To pass properties to a React component we can use this syntax:
The Panel
component will receive these properties in the form of an object (usually named as props
) and they will be accessible inside it through the syntax props.propertyName
:
Below is the updated version of the Panel
component, which dynamically displays a unique title for each instance.
- Each instance of the
Panel
component in the App
component is now passed a unique title
prop (e.g., title="one"
, title="two"
, title="three"
).
- These props allow each
Panel
component to render different content based on the value of the title
.
# TypeScript and Properties
The problem of the previous example is that the props
object is not typed, so:
-
When we open the source code of Panel
in the future we will not know at a glance which properties it supports.
We will have to analyze the code and template inside it and understand it.
Obviously not a problem now that the component is simple, but it becomes difficult when the component starts to be more complex.
-
Even when we instantiate the Panel
component we will definitely not remember which properties it supports.
There would be a way to quickly document our component.
-
We may pass wrong properties and will not have any error notification, except at runtime, when running
TypeScript helps us solve all these problems.
We can type the props object by defining which properties it supports and what type they accept
In the following example we create a new PanelProps
type and we use it to type the props
of the component.
- TypeScript will suggest all available properties.
For example, PanelProps
in the snippet below contains title
and description
, so both properties are suggested:
The description
property is added only for demonstration purposes even if it is not used in our code
- We will have error notifications if we pass properties that don't exist or with a value that has the wrong type:
- Many editors/ IDEs provide suggestions of supported properties already at the moment we create an instance of the component.
In the image below we show the editor that we use on this site, StackBlitz, but many IDE’s like WebStorm and Visual Studio Code work much better:
# Pass Functions as properties
In the previous example we saw how to pass a string
property to the Panel
component.
We can also pass numbers, booleans, complex objects or even functions, as shown in the following example.
Passing a function (as prop) to a component is a technique widely used in React and in many other front-end libraries to abstract the functionality of a component and make it more flexible.
In this way the child component, that is Panel
, will not have to integrate the logic but it will be passed on by the parent, which can decide then its behavior.
We then update Panel
:
- A new button is added in its template
- The type now accept an
onAction
function too
- The parent passes different functions to two Panel instances: in the first case, the button click will display an
alert
, while in the second case a console.log
:
# Pass data from children
Accedi a TUTTI I CORSI PREMIUM ad un unico prezzo!