Work with arrays
Quick guide on JavaScript array methods, immutability and mutability
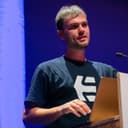
Fabio Biondi
Google Expert | Angular
# Quick introduction
TypeScript Arrays in JavaScript are used to store multiple values within a single variable.
They form an ordered collection of items, in which each item is indexed starting from 0
.
Arrays can hold any data type, including numbers, strings, objects, and even other arrays (known as nested arrays).
# at method
The Array at()
method has been introduced in ECMAScript 2022 (ES13).
So, it is supported starting from Node.js 16 and modern browsers that implement ES2022 or later versions.
The at()
method allows you to access an element of an array using a positive or negative index.
It is particularly useful for accessing the last elements of an array without having to manually calculate the length.
# Add and remove elements: push, pop, splice
Here are examples of how to use push()
, pop()
, and splice()
with the names
array:
# Shift & Unshift
The shift()
method:
1removes the first element from an array
2returns that removed element
This method changes the length of the array.
The unshift()
method:
1adds the specified elements to the beginning of an array
2returns the new length of the array
# forEach
forEach
method allows to iterate over each element in the list
array:
This code defines an array list with values [1, 2, 3, 4, 5]
and uses the forEach
method to loop through each element, executing the provided function for each item, which logs the numbers, 1
, 2
, 3
, etc. to the console.
The following example generates the same result but using arrow functions:
# map
The map()
method creates a new array by applying a provided function to each element of the original array, without modifying the original array.
This example shows how to use the map()
method to create a new array by multiplying each element of the original array (list
) by 2
:
Unlike forEach()
, which only iterates over the array, map()
allocates memory for a new array and returns it.
The original array remains unchanged.
This is important because
map()
always returns a new array, which requires additional memory allocation for storing the transformed values.
This concept will be useful when use immutability, a concept we introduced in the
first lessons of the book and which we will talk about in the following paragraphs.
# filter
The filter()
method creates a new array containing only the elements from the original array that satisfy a provided condition (return true
), without modifying the original array.
The next snippet analyzes element by element and creates a new array in which only values greater than 3
are present:
# Work with an array of objects
This code snippet demonstrates how to use chaining of filter()
and map()
methods to process an array of user objects:
-
filter
: we ensure that users
array returns only the users who are older than 18.
In this case, it filters out Silvia and Fabio, leaving only Lorenzo and Lisa.
-
map
: we create a new array of strings using map
, where each element is the user's name
and age
in the format "name (age)".
For Lorenzo and Lisa, it generates the strings "Lorenzo (26)" and "Lisa (23)".
# find
This code uses the find()
method to search the users
array and returns the first user object where id
is equal to 4
, which is:
{id: 4, name: 'Lisa', age: 23, gender: 'F', city: 'Gorizia'}
:
# findIndex
This code uses the findIndex()
method to search the users
array and returns the index
of the first user whose id
is equal to 4
.
In this case, it would return the index 3
, as the user with id:4
(Lisa) is located at the 4th position (index 3
) in the array:
# some
This code uses the some()
method to check if there is at least one user in the users array whose gender is M
.
It returns true
if there is at least one male (M
) user; otherwise, it returns false
.
In this case, since there are male users (Fabio and Lorenzo), it would return true
.
# every
This code uses the every()
method to check if all users in the users
array have a gender of F
.
It returns true
only if every user in the array is female (F
), otherwise, it returns false
.
In this case, since not all users are female (Fabio and Lorenzo are men), it would return false
.
# React Example
This example, built in React, shows how can we display a list of users
using the map
array method.
Not only that, first we filter the list to show only users over 18 years old.
# Crud Example (Mutability approach)
Let’s see an example where we simulate client-side CRUD operations in which we insert, delete and update elements from a user array:
Accedi a TUTTI I CORSI PREMIUM ad un unico prezzo!