Components
Organize your views in components
And create reusable components using Signal Inputs and Content Projection

v.18
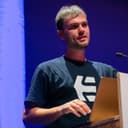
Fabio Biondi
Google Expert | Angular
The goal of this chapter is to start exploring the world of components.
We will discover how to organize a page into components to make the application easier to update, apply the Single Responsibility Principle, create reusable and smart components.
1Learn how convert static HTML template in components
2Create reusable component using the latest Angular (v.18) features and API
3Create a dynamic UI from JSON
# Introduction
As developers, we're always looking for ways to build efficient, scalable, and maintainable applications.
One of the key strategies to obtain these features is using grain fine components
Components are the building blocks of modern web development frameworks like Angular, React, and Vue.
They allow us to create applications that are not only robust but also easy to manage and expand.
In this chapter we'll explore the benefits of using components, focusing on concepts like splitting views, adhering to the Single Responsibility Principle, and reusability.
Split Views for Better Organization
Imagine trying to build a house without any rooms. It would be chaotic, right?
Similarly, in web development splitting views into components help organizing the structure of your application.
Each component represents a distinct part of the user interface (UI).
By dividing your application into components, you can separate different functionalities into distinct, manageable pieces.
For instance, in an e-commerce site, you might have components for the product list, shopping cart, and user profile.
This separation makes easier to focus on each part without getting overwhelmed by the entire application.
When views are split into components, the code becomes more readable.
Each component has its own template, styles, and logic, making it clear what each part of the UI is supposed to do.
# Single Responsibility Principle (SRP)
The Single Responsibility Principle is a foundation of software development, and it states that a class or module should have only one reason to change. Applying SRP to components means that each component should have a single, well-defined purpose.
-
Focused Functionality: when a component is responsible for just one thing, it's easier to understand and maintain. For example, a UserProfile component should only handle displaying and editing user information, not managing user authentication.
-
Easier Debugging: If something goes wrong, knowing that each component has a single responsibility makes it easier to pinpoint where the issue might be. This focused approach reduces the complexity of debugging.
# Reusability Across the Application
One of the most powerful aspects of components is their reusability.
Once you've created a component, you can use it in multiple places within your application.
-
Consistent UI: reusing components ensures a consistent look and feel across your application. For example, if you have a Button component, using it everywhere guarantees that all buttons look and behave in the same way.
-
Efficiency in Development: reusable components save development time. Instead of writing the same code repeatedly, you write it once and reuse it. This not only speeds up development but also reduces the chances of bugs.
-
Easy Updates: when you need to make changes, updating a reusable component updates it across the entire application. This centralized control makes maintaining and evolving your app much simpler.
# Improved Testability
Components, by their nature, are modular and self-contained. This modularity makes it easier to write unit tests for individual components.
-
Isolated Testing: since components encapsulate their functionality, you can test them in isolation. This means you can ensure each component works correctly on its own before integrating it into larger parts of the application.
-
Mocking Dependencies: components often rely on external services or data. With isolated components, it's easier to mock these dependencies, allowing for more comprehensive and controlled testing scenarios.
# In this chapter
1. From Static to Component
This chapter begins by replacing the static Tailwind layout with one that makes use of a more modular system made of static components
We'll split the layout in simple multiple components, and each one will contain the part of the HTML template it is responsible for.
Then we'll convert components in reusable components that you might use everywhere in your current project (or in other projects)
The last step is the creation of the whole UI from a JSON, creating a dynamic page
We will take a static layout created in Tailwind & Daisy UI:
...and we will convert it to a dynamic layout composed by reusable components
Accedi a TUTTI I CORSI PREMIUM ad un unico prezzo!